Build MySQL Queries the OOP Way
and for the composer crowd
"require":{ "davewid/peyote": "0.6.*" }
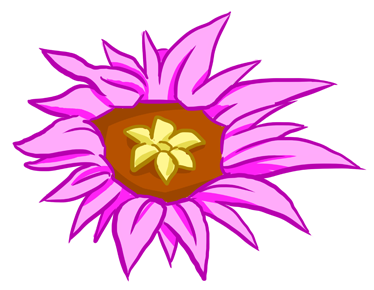
Easy to Learn
Build database queries in an easy to understand and highly reusable way.
Open Source
Peyote is licensed under the fairly open MIT license. Use the library as you wish!
Example
// Create a PDO instance $pdo = new PDO($dsn, $user, $password); // Create a SELECT query $query = new \Peyote\Select('user'); $query->where('user_id', '=', 1); // Build the PDOStatement $statement = $pdo->prepare($query->compile()); // Run the query $statement->execute($query->getParams()); // Fetch results $results = $statement->fetchAll();
Select
$data = array( 'email' => "testing@foo.com", 'password' => "youllneverguess" ); $query = new \Peyote\Insert('user'); $query->columns(array_keys($data))->values(array_values($data)); echo $query->compile(); // INSERT INTO user (email, password) VALUES (?, ?)
Insert
$data = array( 'email' => "testing@foo.com", 'password' => "youllneverguess" ); $query = new \Peyote\Insert('user'); $query->columns(array_keys($data))->values(array_values($data)); echo $query->compile(); // INSERT INTO user (email, password) VALUES (?, ?)
Update
$data = array( 'password' => "iguesssomebodyguessed" ); $query = new \Peyote\Update('user'); $query->set($data)->where('user_id', '=', 1); echo $query->compile(); // UPDATE user SET password = ? WHERE user_id = ?
Delete
$query = new \Peyote\Delete('user'); $query->where('user_id', '=', 1); echo $query->compile(); // DELETE FROM user WHERE user_id = ?